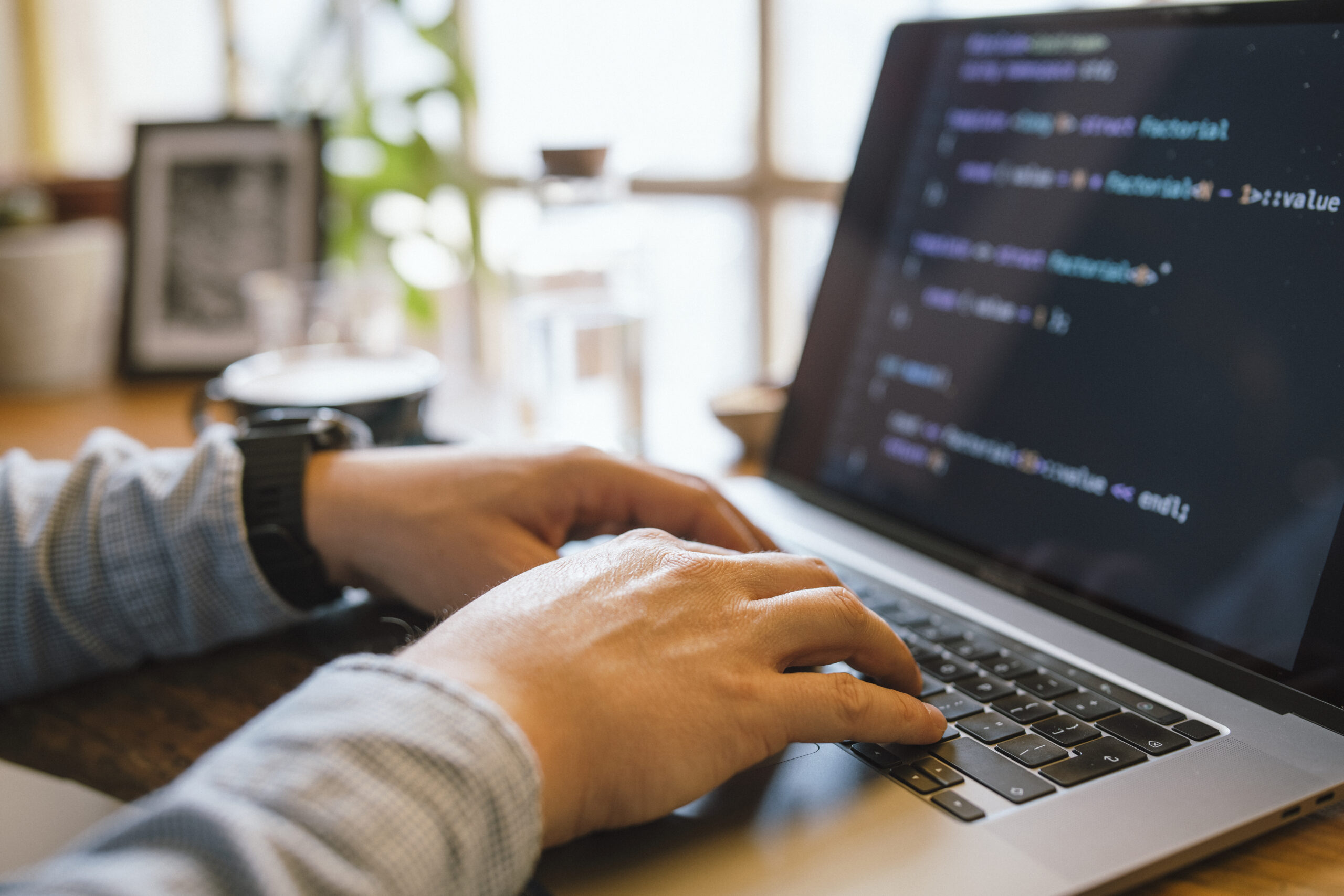
Debugging is Just about the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It's not just about repairing damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Believe methodically to unravel challenges competently. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and drastically boost your productivity. Allow me to share many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use daily. Whilst writing code is a person Component of growth, realizing the way to interact with it correctly through execution is equally important. Fashionable enhancement environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface area of what these resources can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, as well as modify code over the fly. When employed correctly, they Enable you to observe just how your code behaves during execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-close developers. They enable you to inspect the DOM, keep an eye on network requests, view genuine-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can transform annoying UI problems into workable responsibilities.
For backend or program-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command above operating procedures and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfortable with Edition Management units like Git to grasp code record, find the exact second bugs have been launched, and isolate problematic improvements.
In the end, mastering your equipment suggests likely outside of default configurations and shortcuts — it’s about acquiring an intimate understanding of your advancement setting making sure that when challenges crop up, you’re not shed at midnight. The better you understand your equipment, the more time you'll be able to devote solving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping in the code or earning guesses, builders need to have to create a consistent ecosystem or state of affairs the place the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Check with issues like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations less than which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical consumer interactions, or mimicking process states. If the issue seems intermittently, think about producing automated exams that replicate the sting cases or condition transitions involved. These exams don't just assist expose the challenge but also avoid regressions Sooner or later.
At times, The difficulty may be setting-specific — it would transpire only on sure working units, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mindset. It demands persistence, observation, plus a methodical solution. But once you can constantly recreate the bug, you are previously halfway to repairing it. By using a reproducible circumstance, you can use your debugging resources much more efficiently, examination prospective fixes securely, and talk additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Improper. As opposed to seeing them as frustrating interruptions, builders really should understand to take care of error messages as direct communications from the process. They generally let you know precisely what happened, exactly where it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the concept meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and quickly start earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root result in. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them 1st.
Crack the error down into pieces. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it point to a particular file and line selection? What module or operate triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Mastering to acknowledge these can dramatically increase your debugging procedure.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context in which the error happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, encouraging you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you understand what’s taking place under the hood without needing to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what degree. Frequent logging amounts consist of DEBUG, INFO, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic details through growth, Data for common occasions (like successful get started-ups), Alert for likely concerns that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your method. Deal with critical activities, state improvements, enter/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, request IDs, and function names, so it’s easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Furthermore, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your applications, and improve the Total maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully discover and fix bugs, developers need to tactic the procedure similar to a detective solving a mystery. This attitude will help stop working complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you'll be able to with no jumping to conclusions. Use logs, examination circumstances, and consumer reviews to piece with each other a clear picture of what’s going on.
Subsequent, form hypotheses. Ask yourself: What can be producing this actions? Have any improvements just lately been manufactured for the codebase? Has this problem occurred prior to less than identical situation? The aim is always to narrow down alternatives and discover prospective culprits.
Then, examination your theories systematically. Make an effort to recreate the problem inside of a managed surroundings. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the results direct you closer to the reality.
Spend shut consideration to little details. Bugs generally conceal during the minimum envisioned spots—like a missing semicolon, an off-by-one error, or a race ailment. Be comprehensive and patient, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes may conceal the actual dilemma, only for it to resurface afterwards.
And finally, preserve notes on Anything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help you save time for long run difficulties and assist Other folks understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be more effective at uncovering hidden troubles in elaborate methods.
Publish Assessments
Crafting tests is one of the best strategies to help your debugging capabilities and Over-all development efficiency. Tests not just support capture bugs early and also function a security Web that offers you self-confidence when producing alterations on your codebase. A very well-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when an issue occurs.
Start with unit tests, which focus on individual functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected piece of logic is working as envisioned. Any time a exam fails, you promptly know wherever to seem, drastically lowering time spent debugging. Device tests are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and close-to-conclusion assessments into your workflow. These aid make sure that various portions of your application work alongside one another efficiently. They’re especially practical for catching bugs that come about in sophisticated systems with multiple parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to definitely Consider critically about your code. To check a characteristic thoroughly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, you may focus on repairing the bug and enjoy your test go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Answer following Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from a new standpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours before. During this point out, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging sessions. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all over, stretch, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really in fact leads to more quickly and more practical debugging Over time.
Briefly, taking breaks is just not an indication of weakness—it’s a wise technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to mirror and assess what went wrong.
Begin by asking your self a number of essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it have been caught earlier with much better methods like unit testing, code critiques, or logging? The solutions typically expose blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll begin to see patterns—recurring problems or common issues—you could proactively prevent.
In crew environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates more info a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as important aspects of your growth journey. After all, many of the very best builders aren't those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer in your ability set. So following time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at what you do.